Sweet, this should be pretty fun, first I started by creating the new folder at C:\Shared\UserProfiles on the Server 2012 R2 box. For this I used the New-Item cmdlet as shown below.
001
|
New-Item -ItemType Directory -Path C:\Shared\UserProfiles -Verbose
|
After Creating the new directory its ready to be shared, I have to say I really utilize PowerShell_ISE.exe and tab completion every time I create a new SMB or Server Message Block share with PSv3.0+
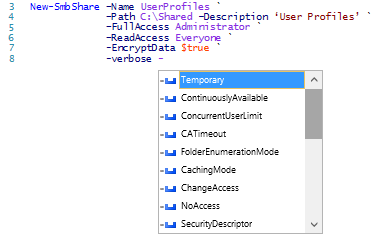
001
002 003 004 005 006 007 |
New-SmbShare –Name UserProfiles `
–Path C:\Shared\UserProfiles ` –Description ‘User Profiles’ ` –FullAccess Administrator ` –ReadAccess Everyone ` -EncryptData $true ` -verbose |
Here is some output: ( Note: the paths are not all aligned as I'm recreating the output from home.)
Next was to copy all of the data from the old server at location A to the new server at location B. For this I used robocopy by opening an administrative CMD prompt and running the below commands :
robocopy \\oldserver\shared \\newserver\shared
001
002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 |
<#
.Synopsis Finds all users with a P: drive as their home drive and resets their homedirectory to equal the New Directory. .DESCRIPTION Retrieves all users with A P: drive and then sets their Home Directory to whatever is specified. .EXAMPLE Set-HomeDirectory .NOTES **New HomeDirectory is hardcoded** .FUNCTIONALITY PowerShell v3.0+ .EXAMPLE PS C:\> Set-HomeDirectory -HomeDrive P: .CREATED BY Matthew A. Kerfoot on 8/12/2014 #> [CmdletBinding()] param([Parameter( Mandatory = $False, ValueFromPipelineByPropertyName = $true )] $Share = "\\SERVERNAME\Shared", [Parameter( Mandatory = $False, ValueFromPipelineByPropertyName = $true )] $HomeDrive = "P:", [Parameter( Mandatory = $False, ValueFromPipelineByPropertyName = $true )] $VerbosePreference = "Continue" ) Begin{ # Find all users with a P: Drive Import-Module ActiveDirectory $users = Get-ADUser -Filter * -Properties * | where {$_.homedrive -eq "P:"} | select -ExpandProperty samaccountname } Process{ # changes each users home drive and homedirectory foreach ($U in $Users) { Set-ADUser -Identity $U -HomeDrive $HomeDrive -HomeDirectory "$Share\$u" -Verbose } } End { # gives a formated output of the changes Get-ADUser -Filter * -Properties * | where {$_.HomeDrive -eq "$HomeDrive"} | Select-Object samaccountname, homedrive, homedirectory ` #| Format-Table -AutoSize } |
Output:
And I just realized the time on this machine is 2 hours behind...
Until next time...